import java.util.ArrayList;
import java.util.HashMap;
public class Solution {
// 양과 늑대
static int maxSheepCnt;
static HashMap<Integer, ArrayList<Integer>> hm;
public static void dfs(int currentIndex, int s, int w, ArrayList<Integer> indexList, int[] info) {
if (info[currentIndex] == 0) {
s += 1;
} else {
w += 1;
}
if (s <= w) return;
maxSheepCnt = Math.max(maxSheepCnt, s);
ArrayList<Integer> nextIndexList = new ArrayList<>();
nextIndexList.addAll(indexList);
if (hm.containsKey(currentIndex)) {
nextIndexList.addAll(hm.get(currentIndex));
}
nextIndexList.remove(Integer.valueOf(currentIndex));
for (int nextIndex : nextIndexList) {
dfs(nextIndex, s, w, nextIndexList, info);
}
return;
}
public static int solution(int[] info, int[][] edges) {
maxSheepCnt = 0;
hm = new HashMap<>();
for (int i = 0; i < edges.length; i++) {
if (!hm.containsKey(edges[i][0])) {
hm.put(edges[i][0], new ArrayList<>());
}
hm.get(edges[i][0]).add(edges[i][1]);
}
// hm : {0=[1, 8], 1=[2, 4], 4=[3, 6], 6=[5], 8=[7, 9], 9=[10, 11]}
ArrayList<Integer> startIndexList = new ArrayList<>();
startIndexList.add(0);
dfs(0, 0, 0, startIndexList, info);
return maxSheepCnt;
}
public static void main(String[] args) {
// TODO Auto-generated method stub
int[] info = {0,0,1,1,1,0,1,0,1,0,1,1};
int[][] edges = {{0,1},{1,2},{1,4},{0,8},{8,7},{9,10},{9,11},{4,3},{6,5},{4,6},{8,9}};
System.out.println(solution(info, edges));
}
}
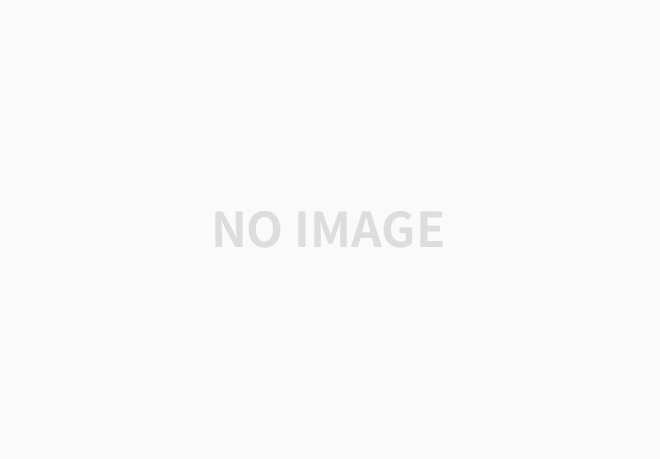
프로그래머스 양과 늑대 문제 풀이 Java
'Java > 프로그래머스' 카테고리의 다른 글
[Java] 프로그래머스 [Level-3] 순위 (0) | 2022.11.29 |
---|---|
[Java] 프로그래머스 [Level-2] 롤케이크 자르기 (0) | 2022.11.29 |
[Java] 프로그래머스 [Level-3] 아이템 줍기 (0) | 2022.11.29 |
[Java] 프로그래머스 [Level-2] 피로도 (0) | 2022.11.29 |
[Java] 프로그래머스 [Level-2] 모음사전 (0) | 2022.11.29 |